Sitecore Headless Services comes out of the box with a powerful Dictionary Service and when using Nextjs/JSS as the technology for your rendering host you’ll get an implementation that interacts with that Dictionary Service API on the Sitecore side. Unfortunately Sitecore isn’t offering this implementation when you’re using the ASP.NET Core Rendering SDK to build out a Sitecore headless front-end rendering host, so you have to implement it yourself.
In this blog post, I’ll guide you through the process of implementing a possible Headless Sitecore Dictionary Service in your ASP.NET Core Rendering Host, leveraging the capabilities of the Sitecore Headless SDK.
Let’s first of all have a look at the Dictionary Service that Sitecore is exposing. Sitecore Headless Services provides an API endpoint for retrieving application-specific Dictionary data and can be accessed at the following endpoint:
The documentation of Sitecore is missing out on the ?sc_apikey= parameter, make sure to add that or else you’ll be thrown an http 400 error. So when accessing this url for example on a project, you’ll get back a JSON response like this (this is the dictionary output out of the box when you’re using the ‘Getting Started template’ to setup your project):
So that’s all great and out-of-the-box coming from Sitecore at the CM/CD side. But like I said, there’s not an out-of-the-box implementation in the headless rendering app with ASP.NET Core when using the ‘Getting Started template’, so we have to implement it ourselves. Let’s have a look at that.
GitHub Kayee Dictionary Service Demo example repository
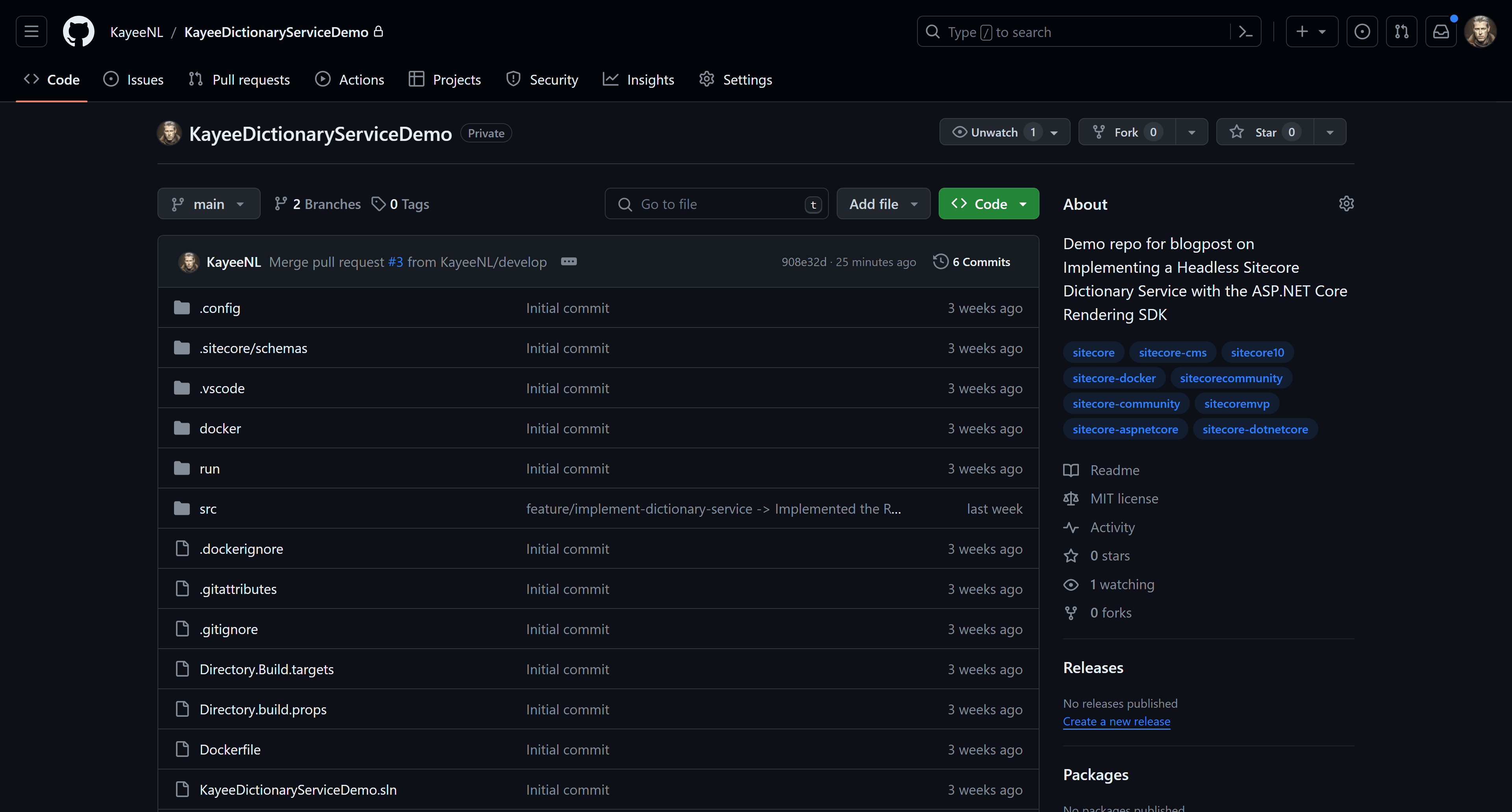
I’ve setup a demo repo on ‘Implementing a Headless Sitecore Dictionary Service with the ASP.NET Core Rendering SDK’. The repo can be found at:
The repository is completely based upon the ‘Getting Started template’, and offers a complete docker based setup, where I’ve implemented the Dictionary Service in the rendering host app. So you’ll be able to check it out and run it yourself. Check out the readme file for instructions. Let’s now walk through the pieces that are part of my implementation. Follow along!
Dictionary Service Options Configuration
When you upon up the Visual Studio solution, you can see that I’ve first defined my configuration in the appsettings.json file
We need to configure the SitecoreInstanceUri first, since this solution is running from inside a container the call from the Rendering Host app back to the Sitecore CM server happens over an internal url, rather than the external url. Hence the configuration is like:
- “SitecoreInstanceUri”: “http://cm/”
When running the Rendering Host from within Visual Studio itself (by pressing F5, it uses the external url for the CM Server), and therefore you then would need to change it to:
- “SitecoreInstanceUri”: “https://cm.kayeedictionaryservicedemo.localhost/”
Next to that we need to configure the API Key, the Sitename and we also provide a caching duration of 15 minutes, the duration for how long the Dictionary Service data will be cached inside the Rendering Host app. More on that later on in this blogpost. Now with the configuration setup let’s have a further look.
I’ve setup a DictionaryServiceOptionsConfiguration class, which basically has properties for the configuration options
IDictionaryService interface
So it basically all comes down to the IDictionaryService interface and in the end the class that actually implements this interface. Let’s delve a little bit deeper into the key components of my ASP.NET Core implementation:
This interface will serve as the blueprint for fetching and managing dictionary data in the Rendering Host application. Now let’s have a look at the implementation.
RestDictionaryService class
As you can see in the RestDictionaryService implementation below the configuration for the DictionaryService is read and used in the various places in the code where it’s needed, until in the end the request is made from within the Rendering Host app to the Dictionary Service endpoint on the Sitecore side.
During initialization, it receives an instance of the IMemoryCache interface, which is crucial for caching data, and a set of configuration options defined in the DictionaryServiceOptionsConfiguration class. Here, the service extracts configuration options, such as the API endpoint and cache duration, from the application configuration. It also obtains an instance of the memory cache for efficient data storage.
The primary function of the RestDictionaryService is to fetch dictionary data. The FetchDictionaryData method is responsible for this task. This method first checks if the required data is already present in the cache. If cached, it returns the data; otherwise, it makes an HTTP request to the Sitecore Dictionary Service API Endpoint using the FetchData method.
Caching plays a crucial role in optimizing the performance of the Rendering Host application. The SetCacheValue method is responsible for storing fetched data in the memory cache. This method sets a cache entry with a specific expiration time (default to 15 minutes), ensuring that the data is refreshed periodically to keep it up-to-date. In the Rendering Host I’ve not bothered with cache clearing upon publishing, this therefore means that if a Content Author updates a dictionary item and publishes, it isn’t immediately updated in the Rendering Host app, after the caching duration expires it will make a new call with the API Endpoint to get the updated phrases from Sitecore.
Dictionary Service Endpoint Response
With that all setup, I’ve also configured a class that can handle the JSON response that’s coming from the API Endpoint, which is used in the above RestDictionaryService class.
DI Service Registration
The primary purpose of DictionaryServiceExtensions class is to simplify the process of configuring dependency injection for the dictionary service. The AddDictionaryService method extends the IServiceCollection interface and registers the RestDictionaryService as an implementation for the IDictionaryService interface.
In the startup class (in Startup.cs), I’m leveraging this extension to enhance the DI container with the Dictionary Service. By invoking the AddDictionaryService method, the application gains access to the dictionary service throughout its components, promoting a clean and modular design.
Wrapping model around the Dictionary Service
The DictionaryModel utilizes lazy initialization and asynchronous fetching, and is introduced to ensure optimal performance when retrieving dictionary data. The constructor accepts an IServiceProvider, enabling dependency injection for accessing the IDictionaryService. The GetDictionaryPhrase method simplifies the process of obtaining translated phrases by key.
See it all in action
In the solution I’ve updated the ContentBlock view. The view utilizes the DictionaryModel to seamlessly integrate dictionary data into the rendering process. By injecting the IServiceProvider and initializing a DictionaryModel instance, we can easily call the GetDictionaryPhrase method to get the translated data from Sitecore. And once executed here’s how it’s displayed

And that’s about it. In case you’re curious or liked this blogpost, make sure to check out the repo and see it in action yourself:
Happy Sitecore-ing!
–Robbert